Meta Data
Within the SCX context, there is no concept of a product catalogue. Only listing data is transmitted via the SCX interface. This can, however, also contain detailed product data, if required by the channel.
A channel must provide descriptive data to describe what a listing may look like on a connected marketplace - we call it Meta Data.
Prices Types
There must be at least one price type available to create a listing on a connected marketplace. Examples of price types are B2C or B2B prices.
Example
// POST https://scx-sbx.api.jtl-software.com/v1/channel/price
{
"priceTypeId": "MarketplaceTypeId",
"displayName": "Marketplace Price",
"description": "Selling price on Marketplace"
}
The priceTypeId
will be transmitted with the Seller:Offer.New
or Seller:Offer.Update
seller events.
{
"sellerId": "1",
"offerId": 4711,
"channelCategoryId": "Stuff",
"quantity": "508.00",
"taxPercent": "19",
"priceList": [
{
"id": "MarketplaceTypeId",
"quantityPriceList": [
{
"amount": "6.95",
"currency": "EUR",
"quantity": "1"
}
]
}
],
"...": "..."
}
Category Tree
A connected channel may provide a category structure to set specific attributes related to a category.
Eexample
The API endpoint is replacing the entire category structure.
// PUT /v1/channel/categories
{
"categoryList": [
{
"categoryId": "1",
"displayName": "First Category",
"listingAllowed": false,
"parentCategoryId": "0"
},
{
"categoryId": "1.1",
"displayName": "First Leaf Category",
"listingAllowed": true,
"parentCategoryId": "1"
},
{
"categoryId": "1.2",
"displayName": "Second Leaf Category",
"listingAllowed": true,
"parentCategoryId": "1"
}
]
}
Attributes
Attributes provide a very simple but powerful way of describing a listing for a channel. This allows the channel to define all marketplace requirements for a listing by means of attributes. SCX differentiates between three types of attributes. Each attribute type shares the same general structure but targets a different use case.
Global Attributes
Global attributes should be used when data is required for each listing.
Category Attributes
Category attributes are related to a categoryId
inside the category structure. JTL-Wawi will display these attributes only when the listing is part of a given category.
Seller Attributes
Seller attributes are global attributes and can be used when a seller requires individual settings for their listings.
Item-specific Attributes
Item-specific attributes are not specified by the channel but are instead created and transferred by the seller. As such, these attributes represent a special type — each attribute must provide a simple, non-schematic, key-value data structure. These attributes will be transmitted along with the channelAttributeList
in each OfferNew or OfferUpdate Event.
These attributes are highly specific and depend on the input of the seller. The channel can use these attributes to provide product and listing data.
Attributes Examples
Attributes with different Types.
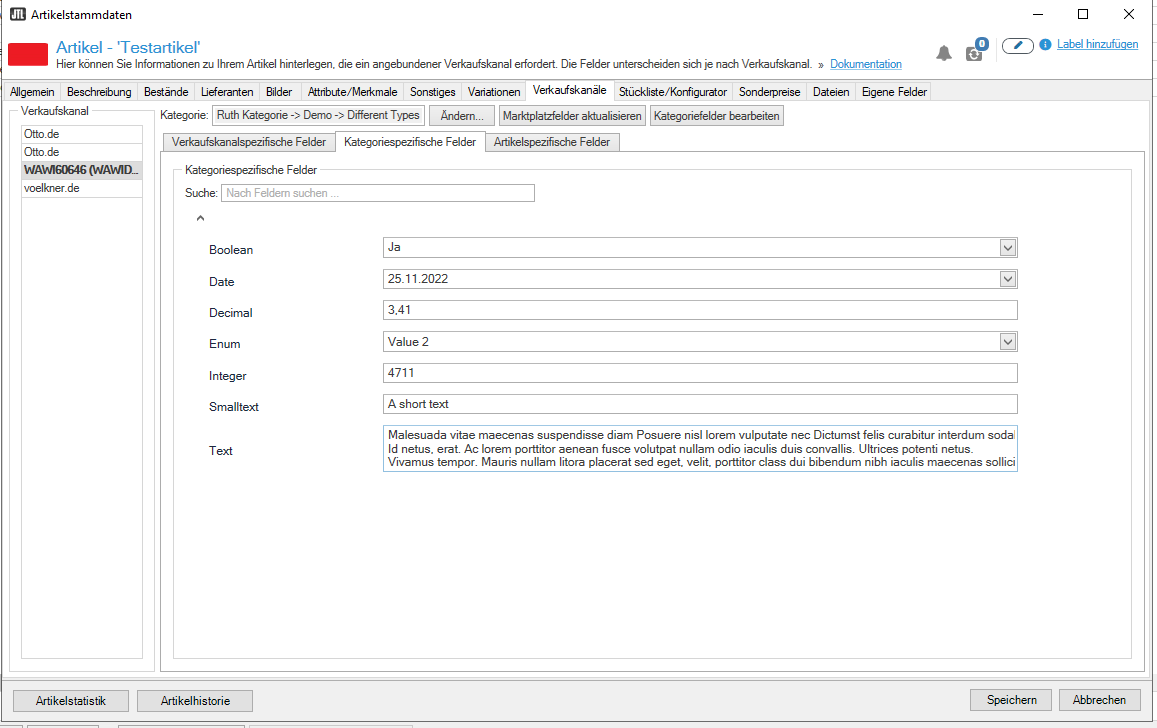
// POST /v1/channel/attribute/category/DEMO-TYPES
{
"attributeList": [
{
"attributeId": "DEMO-TYPES_smalltext",
"displayName": "Smalltext",
"type": "smalltext"
},
{
"attributeId": "DEMO-TYPES_text",
"displayName": "Text",
"type": "text"
},
{
"attributeId": "DEMO-TYPES_integer",
"displayName": "Integer",
"type": "integer"
},
{
"attributeId": "DEMO-TYPES_decimal",
"displayName": "Decimal",
"type": "decimal"
},
{
"attributeId": "DEMO-TYPES_boolean",
"displayName": "Boolean",
"type": "boolean"
},
{
"attributeId": "DEMO-TYPES_enum",
"displayName": "Enum",
"type": "enum",
"values": [
{"display": "Value 1", "value": "Id1", "sort": 10},
{"display": "Value 2", "value": "Id2", "sort": 20},
{"value": "This has no Display"}
]
},
{
"attributeId": "DEMO-TYPES_htmltext",
"displayName": "Html-text",
"type": "htmltext"
},
{
"attributeId": "DEMO-TYPES_date",
"displayName": "Date",
"type": "date"
},
{
"attributeId": "DEMO-TYPES_image",
"displayName": "Image",
"description": "Link to Image file",
"type": "image"
},
{
"attributeId": "DEMO-TYPES_document",
"displayName": "Document",
"description": "Link to a Document file",
"type": "document"
}
]
}
Using sections/sub-sections to organize attributes into logical groups
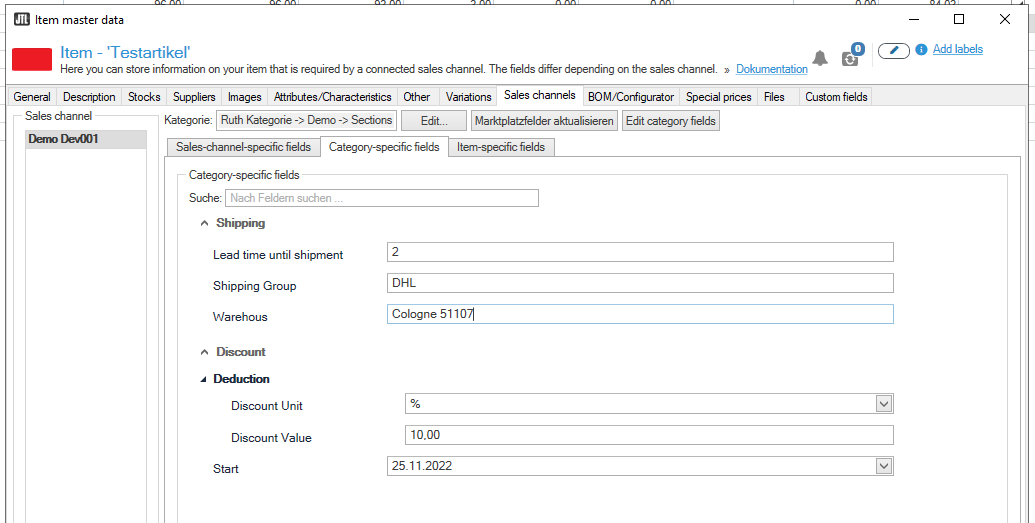
// POST /v1/channel/attribute/category/DEMO-SECTIONS
{
"attributeList": [
{
"attributeId": "DEMO-SECTIONS_WAREHOUS",
"displayName": "Warehous",
"type": "smalltext",
"section": "Shipping",
"sectionPosition": 100
},
{
"attributeId": "DEMO-SECTIONS_SHIPPING",
"displayName": "Shipping Group",
"type": "smalltext",
"section": "Shipping",
"sectionPosition": 80
},
{
"attributeId": "DEMO-SECTIONS_LEADTIME",
"displayName": "Lead time until shipment",
"type": "integer",
"section": "Shipping",
"sectionPosition": 50
},
{
"attributeId": "DEMO-SECTIONS_OFFER_START",
"displayName": "Start",
"type": "date",
"section": "Discount",
"sectionPosition": 50
},
{
"attributeId": "DEMO-SECTIONS_OFFER_DISCOUNT",
"displayName": "Discount Value",
"type": "decimal",
"section": "Discount",
"sectionPosition": 100,
"subSection": "Deduction",
"subSectionPosition": 100
},
{
"attributeId": "DEMO-SECTIONS_OFFER_DISCOUNT_UNIT",
"displayName": "Discount Unit",
"type": "enum",
"values": [
{"value": "%"},
{"value": "EUR"}
],
"section": "Discount",
"sectionPosition": 100,
"subSection": "Deduction",
"subSectionPosition": 90
}
]
}
It is also possible to create repeatable attributes if the attribute supports multiple values.
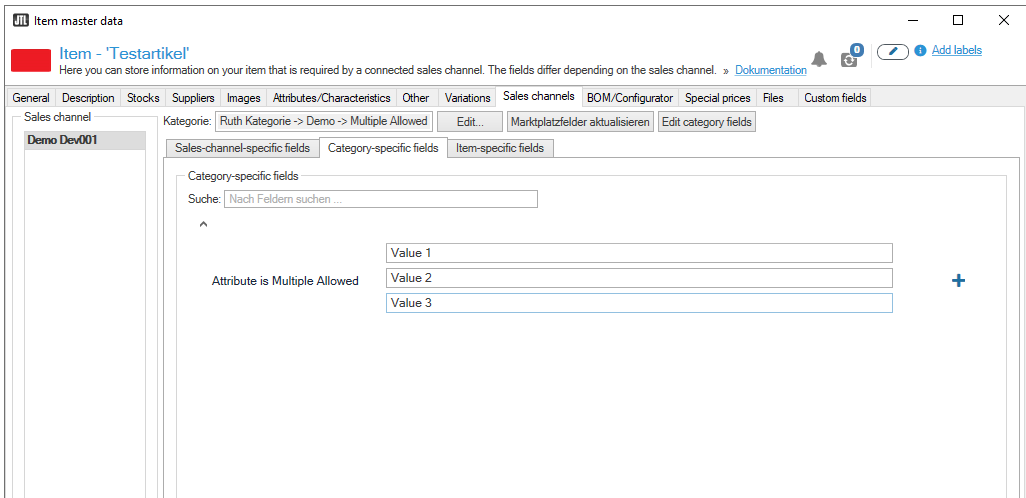
// POST /v1/channel/attribute/category/DEMO-MULTIPLY_ALLOWED
{
"attributeList": [
{
"attributeId": "DEMO-TYPES_isMultipleAllowed",
"displayName": "Attribute is Multiple Allowed",
"isMultipleAllowed": true,
"type": "smalltext"
}
]
}
It is also possible to create repeatable attributes if the attribute supports multiple values.
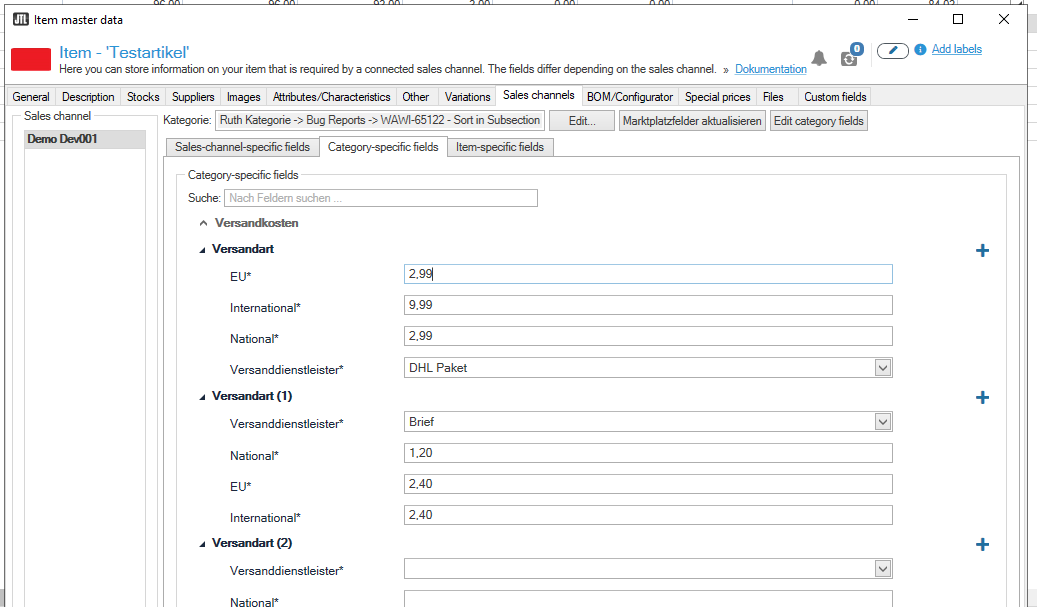
// POST /v1/channel/attribute/category/DEMO-REPEATABLE_SUBSECTIONS
{
"attributeList": [
{
"attributeId": "shipping_carrier",
"displayName": "Versanddienstleister",
"type": "enum",
"values": [
{
"value": "carrierID_2",
"display": "Brief",
"sort": 1
},
{
"value": "carrierID_3",
"display": "DHL Päckchen",
"sort": 2
},
{
"value": "carrierID_4",
"display": "DHL Paket",
"sort": 3
},
{
"value": "carrierID_22",
"display": "Kostenloser Download",
"sort": 20
}
],
"section": "Versandkosten",
"sectionPosition": 2,
"subSection": "Versandart",
"subSectionPosition": 10,
"isRepeatableSubSection": true
},
{
"attributeId": "shipping_cost_nat",
"displayName": "National",
"type": "decimal",
"section": "Versandkosten",
"sectionPosition": 2,
"subSection": "Versandart",
"subSectionPosition": 9,
"isRepeatableSubSection": true
},
{
"attributeId": "shipping_cost_eu",
"displayName": "EU",
"isMultipleAllowed": false,
"type": "decimal",
"section": "Versandkosten",
"sectionPosition": 2,
"subSection": "Versandart",
"subSectionPosition": 8,
"isRepeatableSubSection": true
},
{
"attributeId": "shipping_cost_int",
"displayName": "International",
"isMultipleAllowed": false,
"type": "decimal",
"section": "Versandkosten",
"sectionPosition": 2,
"subSection": "Versandart",
"subSectionPosition": 7,
"isRepeatableSubSection": true
}
]
}
When using repeatable sections, JTL-Wawi generates a group ID, which is then transferred back to the channel with the listing. This makes it possible to recognize related attributes in the listing data.
// GET /v1/channel/event
{
"type": "Seller:Offer.New",
"sellerId": "1",
"offerId": 1,
"channelCategoryId": "DEMO-REPEATABLE_SUBSECTIONS",
"channelAttributeList": [
{
"attributeId": "shipping_carrier",
"value": "DHL Paket",
"group": "1"
},
{
"attributeId": "shipping_cost_nat",
"value": "2.99",
"group": "1"
},
{
"attributeId": "shipping_cost_eu",
"value": "2.99",
"group": "1"
},
{
"attributeId": "shipping_cost_int",
"value": "9.99",
"group": "1"
},
{
"attributeId": "shipping_carrier",
"value": "Brief",
"group": "2"
},
{
"attributeId": "shipping_cost_nat",
"value": "1.20",
"group": "2"
},
{
"attributeId": "shipping_cost_eu",
"value": "2.40",
"group": "2"
},
{
"attributeId": "shipping_cost_int",
"value": "2.40",
"group": "2"
},
]
}