Order Process
- A new order must be created by calling
POST /v1/channel/order
first - The purchase date of an order must be after the creation date of the seller ID
- The orderId is unique, and can only exist once for a seller ID
- It is not possible to add or change order lines once an order has been created.
- Once an order is created, it can be updated using
PUT /v1/channel/order/status
to update the status of an order or order itemPUT /v1/channel/order/address-update
to update the address information
Order Status
CREATED
: Orders with the status “created” are not yet ready for shipping. Orders with this status can be used for stock reservation. Once the order is ready for shipping, the status will change toACCEPTED
.UNACKED
: Orders with this status are not yet ready for shipping. The seller must first accept the order. Note: JTL-Wawi does not yet support order acknowledgment.ACCEPTED
: Orders with the status “accepted” are ready for shipping.
Order line item Status
Each order line item has a status, and this status should be used to determine the order status itself.
UNSHIPPED
: The order line item is ready for shipping and can be shipped once the order isACCEPTED
.SHIPPED
: The order line item is marked as having been shipped.CANCELED_BY_SELLER
: The order line item has been cancelled by the seller.CANCELED_BY_BUYER
: The order line item has been cancelled by the buyer or the marketplace.RETURNED
: The order line item has been returned to the seller.REFUNDED
: The order line item has been refunded.
State transition - limits and rules
- Orders with the statuses
UNACKED
orCREATED
may not have valid address information. - An address update can only apply to orders with the statuses
UNCACKED
orCREATED
. - Address information must be available before a status transition to
ACCEPTED
. - Once an order is assigned the status
ACCEPTED
, the order status cannot be changed.
The order item status can convey the following status information:
Stauts | UNSHIPPED | SHIPPED | CANCELED_BY_SELLER | CANCELED_BY_BUYER | RETURNED | REFUNDED |
---|---|---|---|---|---|---|
UNSHIPPED | ✓ | ✓ | ✓ | ✓ | ✓ | ✓ |
SHIPPED | - | ✓ | ✓ | ✓ | ✓ | ✓ |
CANCELED_BY_SELLER | - | - | ✓ | - | - | - |
CANCELED_BY_BUYER | - | - | - | ✓ | - | - |
RETURNED | - | - | - | - | ✓ | ✓ |
REFUNDED | - | - | - | - | - | ✓ |
Examples
Create a CREATED
order using
// `POST 'https://scx-sbx.api.jtl-software.com/v1/channel/order`
{
"orderList": [
{
"sellerId": "1",
"orderStatus": "CREATED",
"orderId": "OrderId_000001",
"purchasedAt": "2020-02-25T15:05:20+00:00",
"lastChangedAt": "2020-02-25T15:05:20+00:00",
"currency": "EUR",
"orderItem": [
{
"orderItemId": "SHIPPING-0001",
"type": "SHIPPING",
"grossPrice": "2.00",
"taxPercent": "19",
"quantity": "1.0",
"shippingGroup": "test"
},
{
"orderItemId": "ABC-0001",
"type": "ITEM",
"grossPrice": "19.99",
"total": "19.99",
"taxPercent": "19",
"sku": "1234",
"channelOfferId": "1",
"quantity": "1",
"title": "Eine Hose (4006680069951)",
"note": "Zur Auswahl"
},
{
"orderItemId": "ABC-0002",
"type": "ITEM",
"grossPrice": "19.99",
"total":"19.99",
"taxPercent":"19",
"sku": "ART-WAWI-55070",
"channelOfferId": "2",
"quantity": 1,
"title": "Ein Hemd (ART-WAWI-55070)",
"note": "Zur Auswahl"
}
]
}
]
}
Send address information for an order with the status CREATED
// PUT 'https://scx-sbx.api.jtl-software.com/v1/channel/order/address-update
{
"orderList": [
{
"orderId": "OrderId_000001",
"sellerId": "1",
"billingAddress": {
"firstName": "Arno",
"lastName": "Nym",
"gender": "male",
"street": "Am Feld",
"houseNumber": "16",
"postcode": "123456",
"city": "Dingenskirschen",
"country": "DE"
},
"shippingAddress": {
"firstName": "Arno",
"lastName": "Nym",
"gender": "male",
"street": "Am Feld",
"houseNumber": "16",
"postcode": "123456",
"city": "Dingenskirschen",
"country": "DE"
}
}
]
}
Update order and set status to ACCEPTED
// PUT 'https://scx-sbx.api.jtl-software.com/v1/channel/order/status
{
"orderList": [
{
"orderId": "OrderId_000001",
"sellerId": "1",
"orderStatus": "ACCEPTED"
}
]
}
Send a status change for an order line items
// PUT 'https://scx-sbx.api.jtl-software.com/v1/channel/order/status
{
"orderList": [
{
"orderId": "OrderId_000001",
"sellerId": "1",
"orderStatus": "ACCEPTED",
"orderItems": [
{
"orderItemId": "ABC-0001",
"itemStatus": "SHIPPED",
"paymentStatus": "PAID"
},
{
"orderItemId": "ABC-0002",
"itemStatus": "SHIPPED",
"paymentStatus": "PAID"
}
]
}
]
}
Cancellation (by Seller)
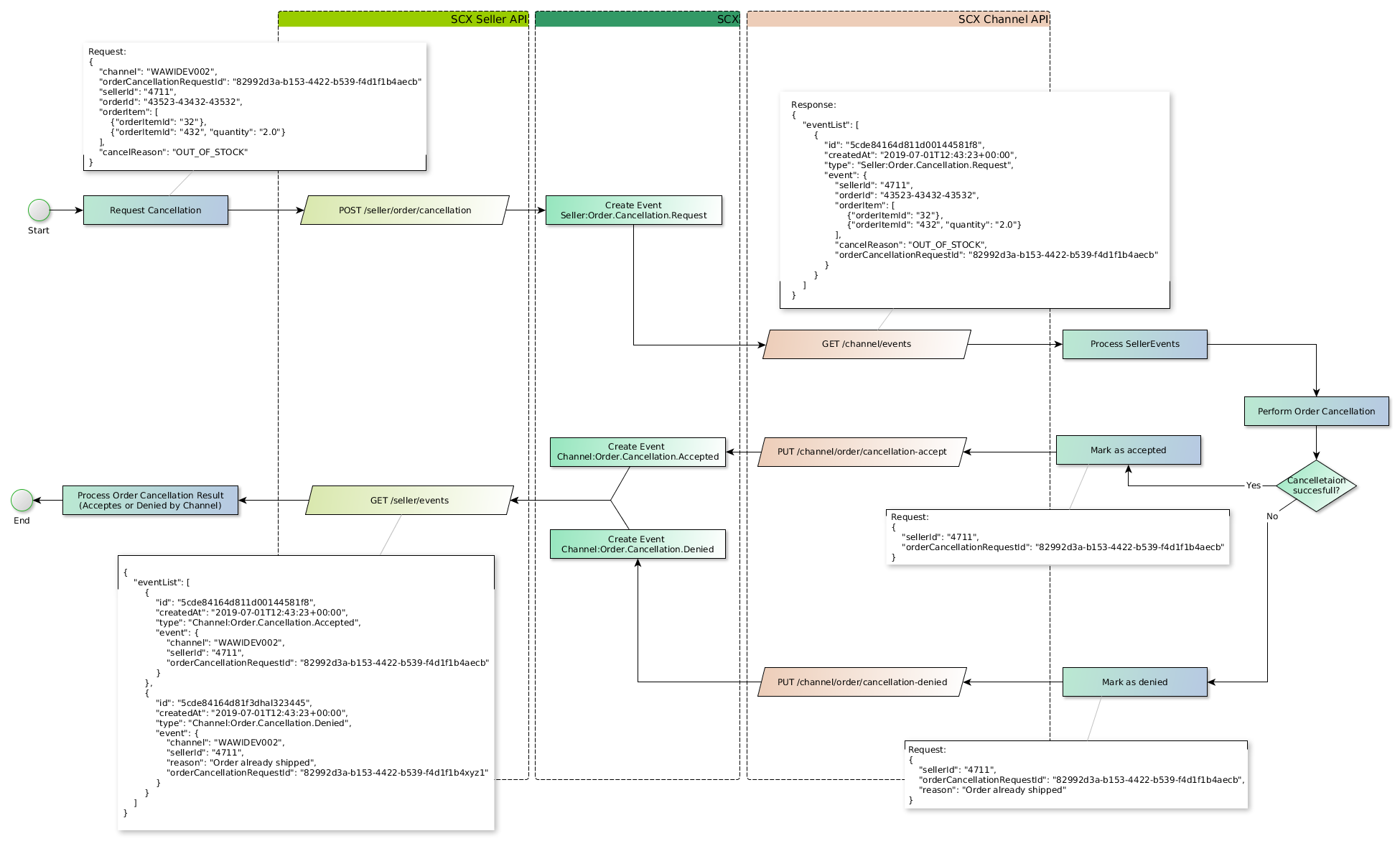
- Sellers send a cancellation request together with a
CancellationRequestId
which the client, i.e., JTL-Wawi, should remember in order to be able to assign the response later on. - The
CancellationRequestId
is used for the unique assignment of the data (OrderId / OrderItemIDs) to the client. - A cancellation should always include all items to be cancelled. If the entire order is cancelled, all order items included in the order must be specified. However, if only part of the order is cancelled, only the cancelled items must be specified.
Cancellation (by Buyer)
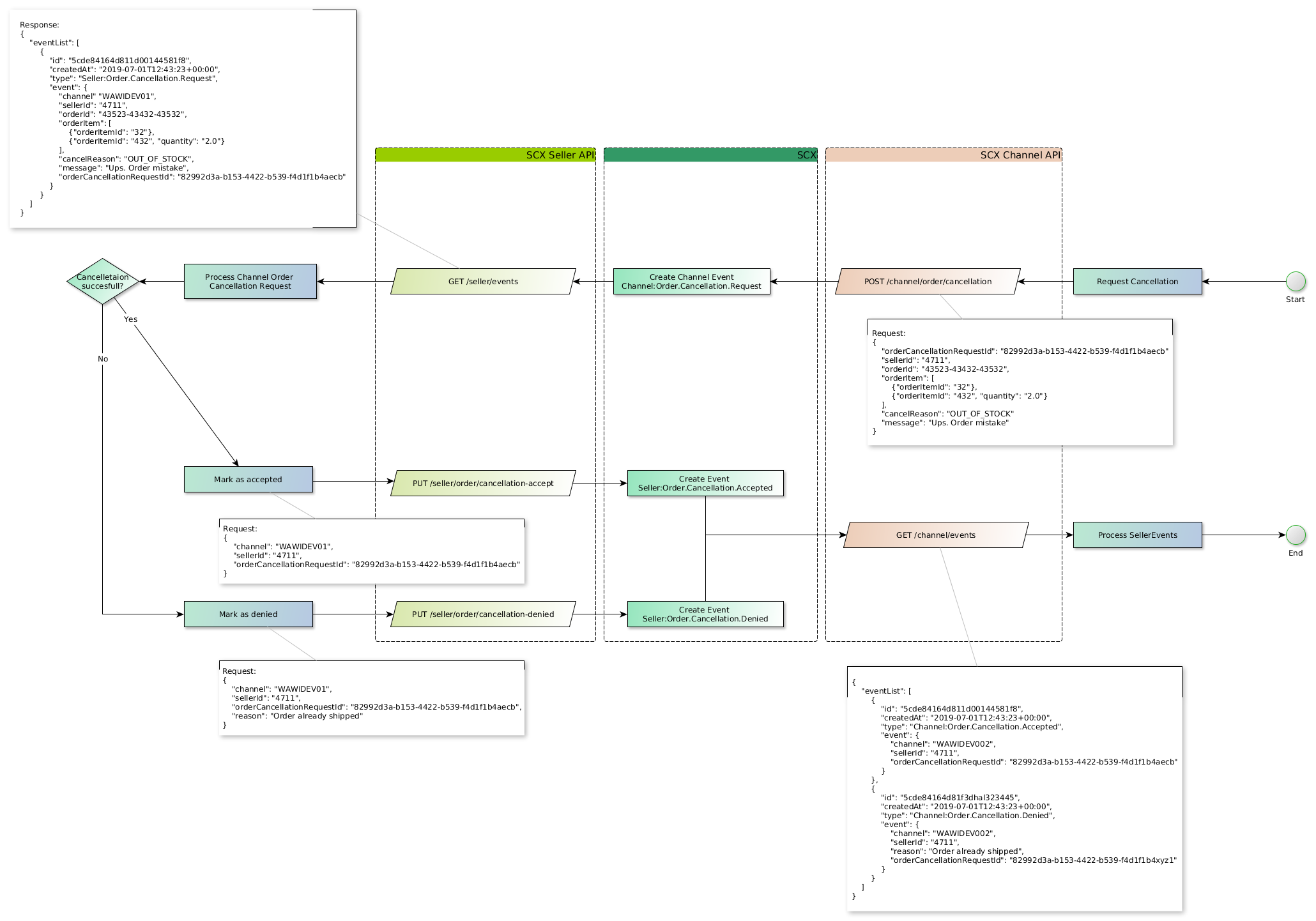
- Buyers or marketplaces send a cancellation request together with a
CancellationRequestId
. - The
CancellationRequestId
is used for the unique assignment of the data (OrderId / OrderItemIDs) to the channel. - A cancellation should always include all items to be cancelled. If the entire order is to be cancelled, all order items contained must also be specified. It is also possible to have partial order cancellations if only some individual items are cancelled.
- Using this workflow on the channel side is considered optional- it is also possible to set the order status to “cancel”.
Return
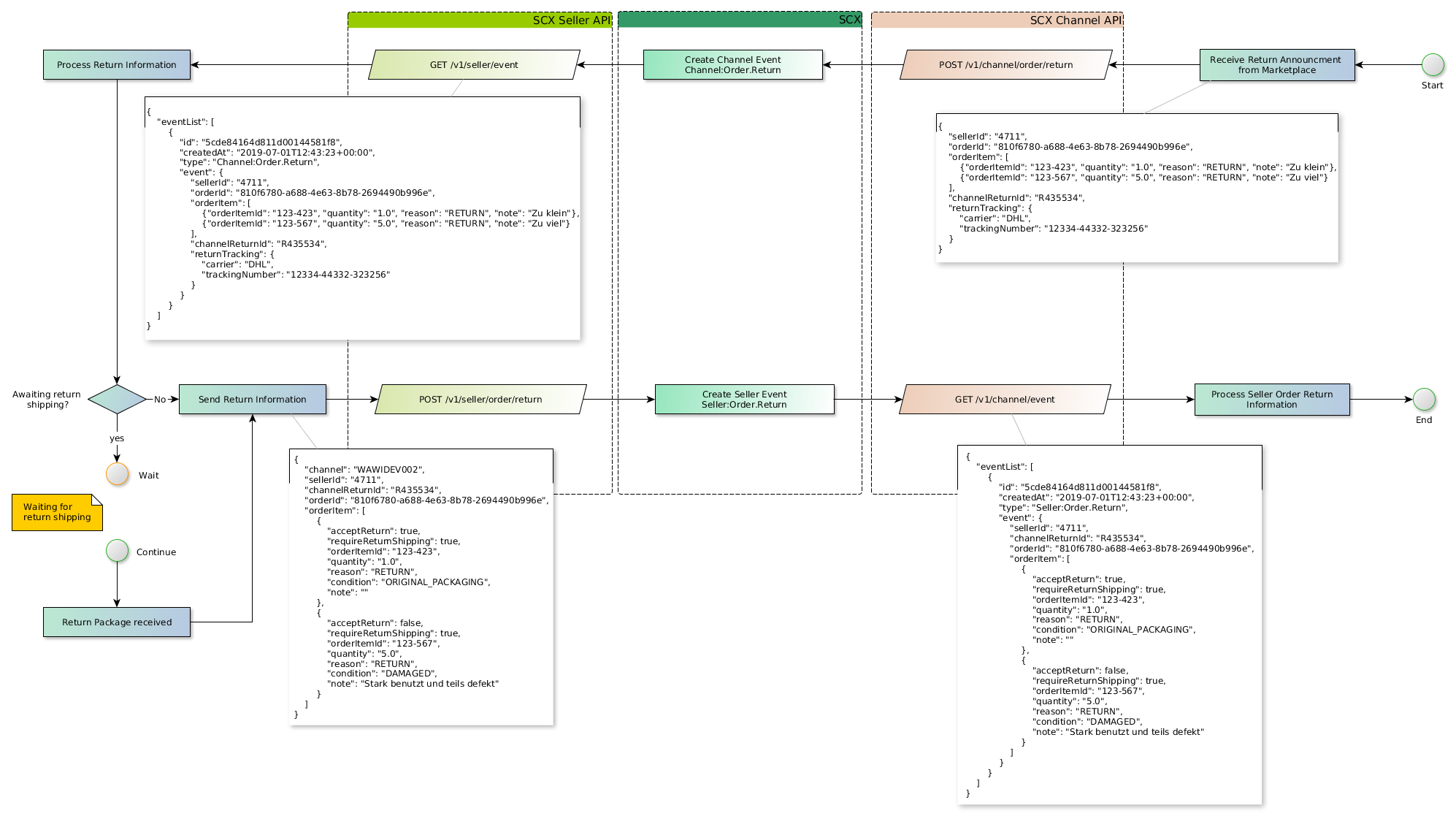
Channel informs about upcoming return event
Note: This is an optional step in the workflow. Not all marketplaces support a return announcement.
- Via the
Channel:Order.Return
event, the channel reports that a return process has been initiated on a connected marketplace.- The following data is provided:
- OrderId
- OrderItem List (ItemId, Quantity, Reason, Comment)
- The following data can be provided optionally by the channel:
- ChannelReturnId - internal ID to identify the return case
- Return Tracking Information (Carrier, TrackingNo.)
- The following data is provided:
- JTL-Wawi stores the upcoming return case
- If no item return is required, the return can be answered directly via
POST /v1/seller/order/return
. - If a return is required, the seller now waits for the incoming return.
- If no item return is required, the return can be answered directly via
(Optional) Merchant decides that no return is required
Note: This is an optional step in the workflow.
The seller has the option to decide whether the customer needs to make a return. If no return is required, the POST /v1/seller/order/return
can be sent by the seller after receiving the Channel:Order.Return event. The property requireReturnShipping
should be set to false
here.
Return shipment arrives at the seller’s warehouse
- Once the return has arrived, the seller will check the items and confirm the return using
POST /v1/seller/order/return
. - If a return already exists in JTL -Wawi, the information stored there should be used to transfer the return to SCX.
- This information includes the
channelReturnId
.
- This information includes the
- Furthermore, the following information about the individual order items must be transferred:
- The order number (orderId)
- The quantity that was returned
- If the return is accepted (acceptReturn)
- A condition must be specified
- A reason can be specified
- An additional note that can be stored
Refund
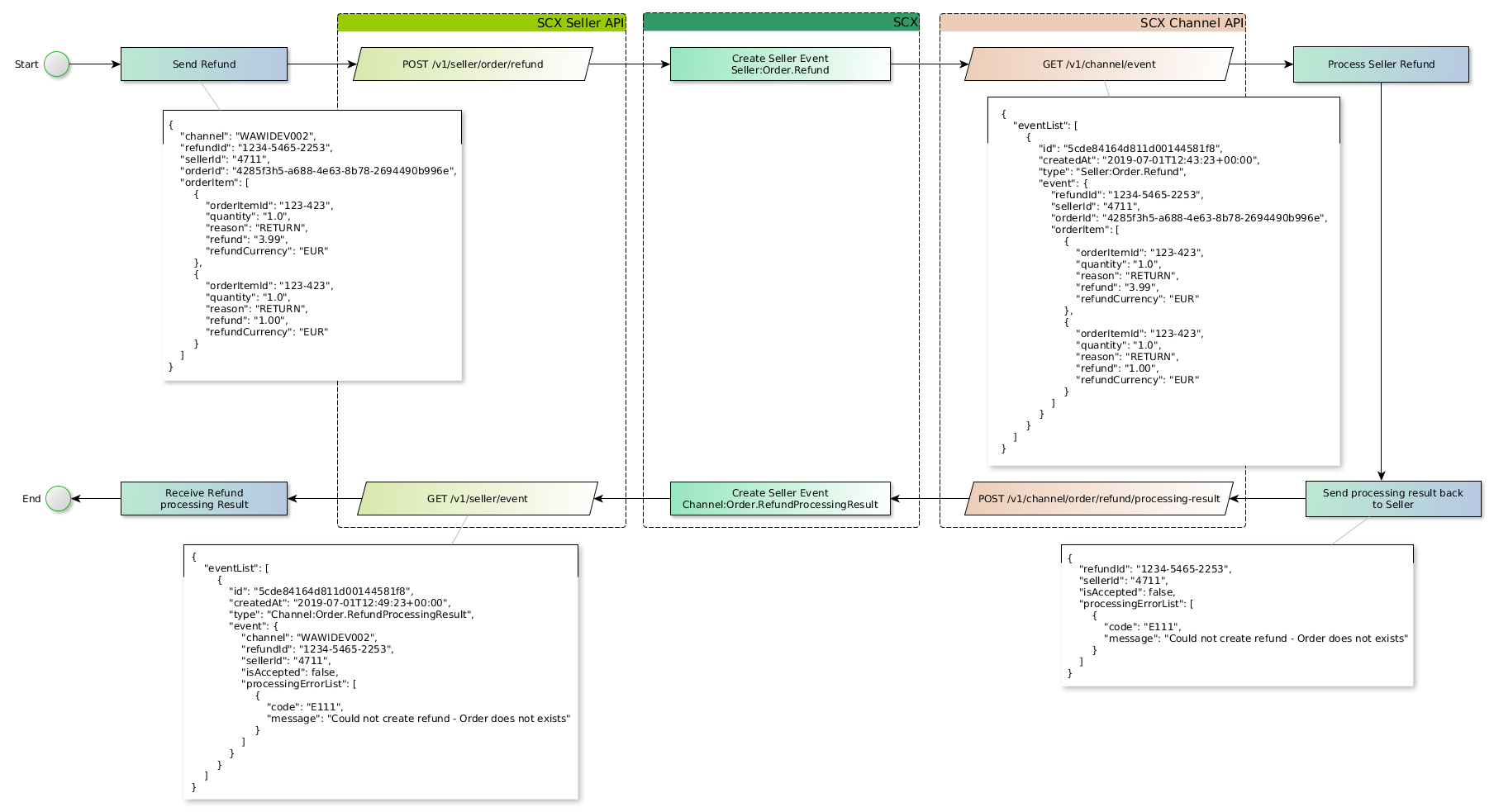
Refunds are initiated directly by the seller, e.g. after a return has been processed or following an agreement with the buyer (via ticket/email/phone). The channel processes the refund and sends a Channel:Order.RefundProcessingResult
after a success or failure back to the seller to ensure that a refund has been properly processed.