Seller Management
A channel must manage seller accounts autonomously. JTL will never be aware of any credentials required by an individual seller to connect to a Marketplace or an external system (including API credentials).
Each channel must maintain a sign-up URL and an update URL. The sign-up URL must point at a login page and the update URL at a sign-up page, both hosted by the channel itself. A seller will create a sign-up or update session in JTL-Wawi. From there, they will be redirected to the sign-up and update URLS hosted by the channel and receive a unique one-time session ID.
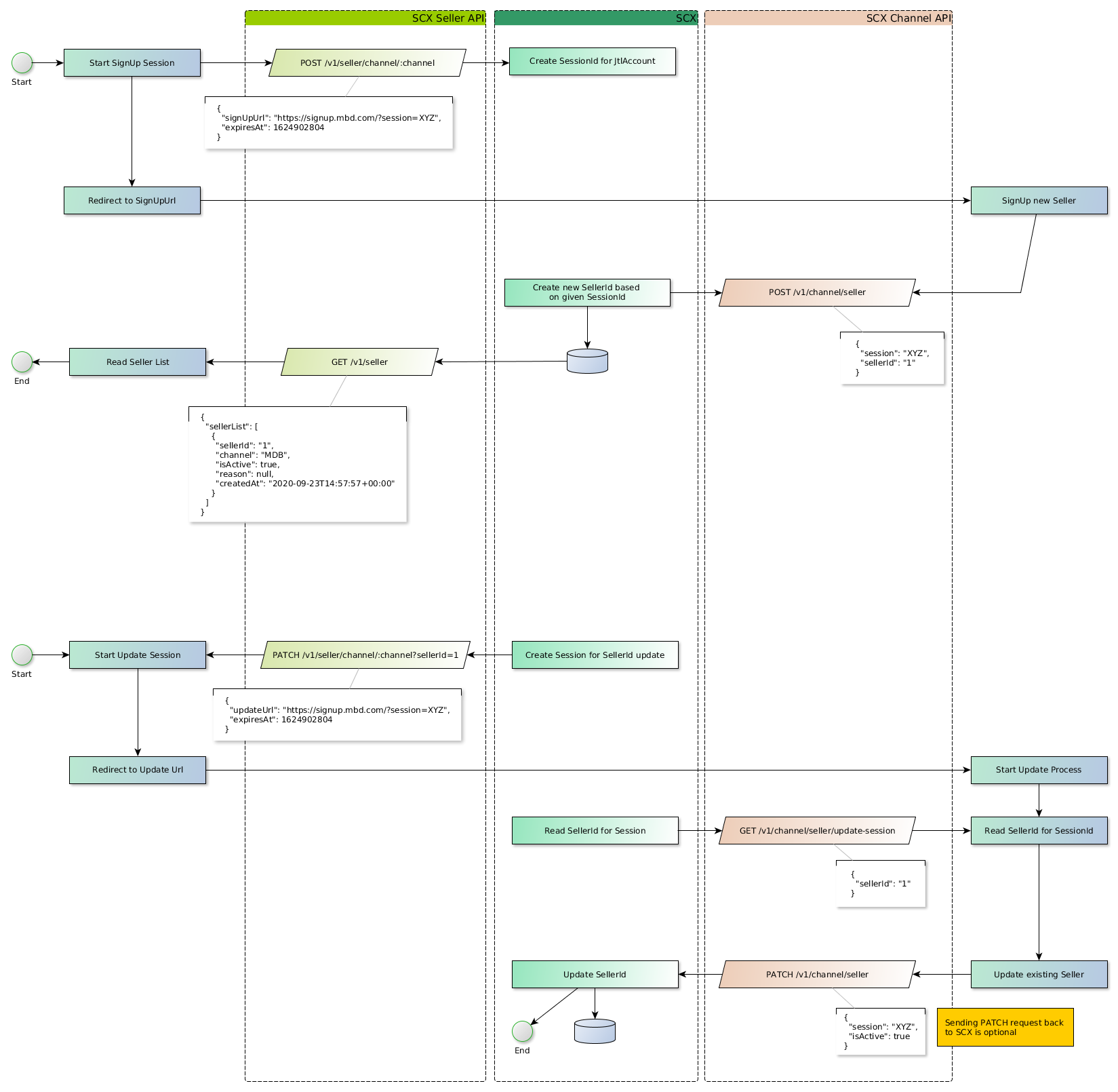
Seller sign-up
The aim of the sign-up process is to avoid managing sensitive access data to a marketplace within the client environment or SCX. The channel itself has sovereignty over the access data to the connected marketplace.
- Channel has a sign-up URL
- A sign-up is initiated via the Seller API
- The seller's JTL-Wawi redirects to the sign-up URL (via web browser).
- The destination of the sign-up URL is a website hosted by the channel. On this website the registration process takes place
- The channel authenticate and stores a new seller and assigns a unique Seller ID.
- The channel reports the generated Seller ID together with the Session ID back to SCX.
- SCX stores the Seller ID to the channel and reports all events with this Seller ID to the channel from now on.
Example
Create a new SignUp Session ID
// POST /v1/seller/channel/MYCHANNEL
{
"signUpUrl": "https://www.mychannel.com/?session=demoSessionId123&expiresAt=1646759360",
"expiresAt": 1646759360
}
Seller is now redirected to the signUpUrl
.
On the sign-up page, the channel must ask for seller identification. If a seller is deemed valid and authenticated, the Channel itself must create a unique Seller ID and send it, along with the Session ID, from the sign-up URL to the Channel API.
Note: All events received from the Channel API will have this Seller ID. This Seller ID is immutable and cannot be changed afterwards.
// POST /v1/channel/seller
{
"session": "demoSessionId123",
"sellerId": "1",
"companyName": "JTL-Software-GmbH"
}
Seller Update
Sometimes, it may be necessary to update the connection between a JTL account and the seller. For example, the access data pertaining to the marketplace may have been changed. In cases like this, the JTL account must be able to update this data on the channel.
- An update URL has been defined for the channel.
- The update process is initiated using the Seller API.
- The client of the JTL account (JTL-Wawi) redirects the user to the update URL.
- The target of the update URL is a website hosted by the channel. On this website the update process takes place.
- The channel asks SCX which seller ID belongs to the current update Session ID.
- The channel enables an update of the seller account.
- After the update process, the channel can also update the seller on the SCX system.
Examples
Seller update process in initiated by creating an update URL for the Channel
// PATCH /v1/seller/channel/MYCHANNEL?sellerId=1
{
"updateUrl": "https://www.mychannel.com/update?session=demoUpdateSessionId123&expiresAt=1646759360",
"expiresAt": 1646759360
}
The seller is then redirected to the update URL by the channel and receives a one-time session ID.
For security reasons, the seller ID is not part of the update URL and must be received through a separate call.
// GET /v1/channel/seller/update-session?sessionId=demoUpdateSessionId123
{
"sellerId": "1"
}
After the update workflow is handed, the Channel may now update the Seller at Channel API.
// PATCH /v1/channel/seller
{
"sessionId": "demoUpdateSessionId123",
"isActive": true,
"companyName": "JTL-Software-GmbH"
}
Seller unlink
There are different scenarios when a Seller reach a state where he is forbidden to use a Channel.
- Sellers deactivate the connection on their own behalf.
- Seller does not have an active JTL-eazyAuction subscription to use the SCX system.
In this scenario, as the channel, you receive a Seller:Channel.Unlinked
event.
{
"sellerId": "1",
"reason": "deactivated by Seller",
"unlinkedAt": "2024-02-13T06:00:00+0000",
"permanentlyRemoved": false
}
We recommend that you deactivate a Seller in your system. SCX will not accept any more data for that seller ID until the seller initiates a seller update process as described above. You should not delete a Seller ID from your system if permanentlyRemoved
is set to false
.
When the permanentlyRemoved
property is set to true
, the Seller ID is completely removed from the SCX system and is no longer known, so you can delete the Seller ID on your end as well.
There are scenarios where you need to unlink a seller ID on your behalf.
- API credentials become invalid or are revoked, requiring you to reauthorize the Seller ID
- Seller does not have an active contract or subscription with your company
- There is suspicious behavior on your end, and you want to stop the seller's activity.
You can do that by sending a DELETE /v1/channel/sellerId/{sellerId}
request. Do not delete the seller ID in your system until you receive a Seller:Channel.Unlinked
event with "permanentlyRemoved": true
.